Handle clicks and display results
In a FastTrack-enabled search application, when a search result is clicked, the properties from the search result document can be displayed. To do this, In the search application, open the App.jsx
file and replace the existing code with this code:
import React from 'react' import './App.css' import { useContext, useState } from "react"; import { MarkLogicContext, SearchBox, ResultsSnippet, WindowCard, EntityRecord } from "ml-fasttrack"; function App() { const context = useContext(MarkLogicContext); const [showWindow, setShowWindow] = useState(false); const handleSearch = (params) => { context.setQtext(params?.q); } const handleResultClick = (snippet) => { context.getDocument(snippet.uri).then((response) => { setShowWindow(true); }) } const handleWindowClose = () => { context.setDocumentResponse(null); showWindow && setShowWindow(null); } return ( <div className="App"> <div> <SearchBox onSearch={handleSearch}/> </div> <div> <ResultsSnippet results={context.searchResponse.results} onClick={handleResultClick} /> </div> <div> <WindowCard title="Person Details" visible={showWindow} toggleDialog={handleWindowClose} initialLeft={640} height={240} width={320} > <EntityRecord entity={context.documentResponse} config={{ entityTypeConfig: { "path": "data.envelope.entityType" }, entities: [ { entityType: 'person', items: [ { label: 'First Name', path: 'data.envelope.firstName' }, { label: 'Last Name', path: 'data.envelope.lastName' }, { label: 'Title', path: 'data.envelope.title' }, { label: 'DOB', path: 'data.envelope.dob' } ] } ] }} /> </WindowCard> </div> </div> ) } export default App
Code explanation
The code in Handle clicks and display results performs these functions:
Adds the WindowCard widget to display the search result details.
The visibility of the window is handled by the state variable
showWindow
. The variable is initially set tofalse
.The callback prop
toggleDialog
handles closing the window. Other props set the window's title, dimensions, and placement in the browser.
Adds the EntityRecord widget inside the WindowCard widget to display the document information.
The
entity
prop sets the retrieved document data (which is stored in the context after retrieval).The
config
prop specifies the document properties to display.See the EntityRecord documentation for more information about configuration.
Adds an onClick
callback prop to the ResultsSnippet widget to handle search result clicks:
The clicked search result is passed to the callback function.
The callback function retrieves the corresponding document using the
getDocument()
method from the context and the document URI from the search result.After the document is successfully retrieved, the callback sets
showWindow
totrue
to open the window.
Rendered result
When a search result is clicked, a window opens and displays the search result content.
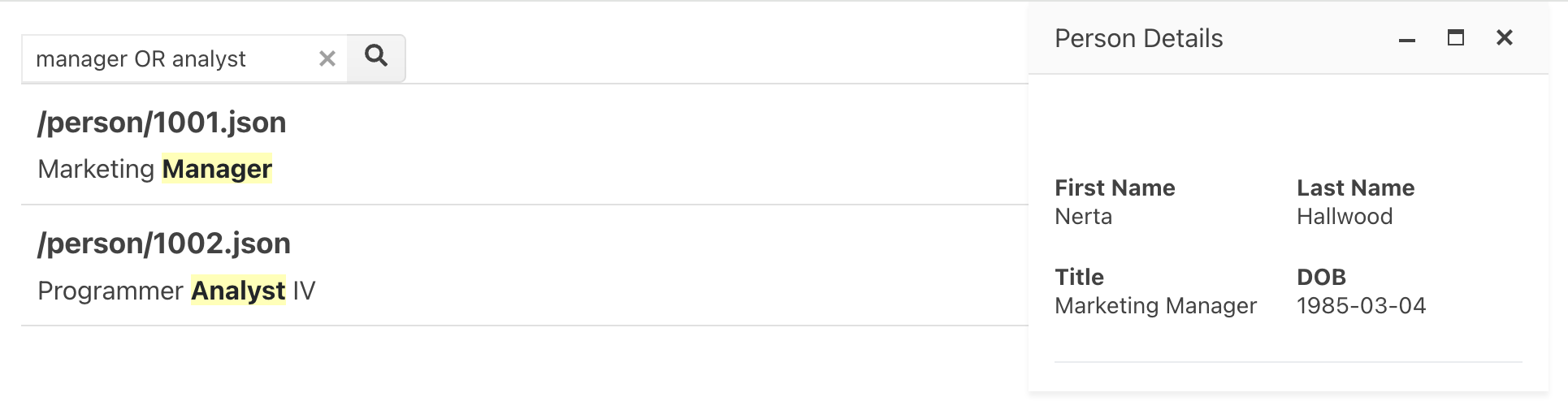