To Insert Documents
You can insert multiple documents at a time--assigning URIs, providing the document data, assigning permissions, and adding the document to various collections among other actions--with a single tidy Optic statement.
Our company was acquired by a larger company. So, we need to add two new departments to our HR documents. Unlike the five original departments, these new departments are temporary and will contain employees that also remain members of their original departments:
The Integration Department contains employees from our original company selected to work with the new company during transition.
The Attrition Department contains employees considered potentially redundant as the integration moves forward.
To add these new department documents, we need to provide what are called document descriptors along with the document itself. In our case, we will include three: a unique URI
for the document, any collections
the document will belong to, and proper permissions
.
An Optic update like this one creates documents with these properties:
declareUpdate(); const op = require("/MarkLogic/optic"); const collections = ["https://example.com/content/department"]; const permissions = xdmp.defaultPermissions(); op.fromDocDescriptors([ { uri: "/data/departments/Integration.json", doc: { "Department": "Integration", "LineOfBusiness": "Cross-Department Collaboration", "Description": "The Integration Department helps the new company determine optimal allocation of resources and employees moving forward." }, collections, permissions }, { uri: "/data/departments/Attrition.json", doc: { "Department": "Attrition", "LineOfBusiness": "Cross-Department Collaboration", "Description": "The Attrition Department contains potentially redundant employees identified as the integration process progresses." }, collections, permissions }]) .write() .execute();
We used this update to add our 2 new departments:
The Data Accessor Function
fromDocDescriptors()
creates 2 4-column rows holding our new documents' URIs, data, collections, and permissions. We could also have provided these document descriptor columns:metadata
quality
temporalCollection
The Operator Function
write()
inserts each document, by default giving it the URI in theuri
column created by the latest data accessor function.The Executor Function
execute()
executes the update without returning any rows.To test the update before writing the document, replace
write()
andexecute()
withresult()
to return the rows thatfromDocDescriptors()
has rendered.
The two new departments take their places among the others in our department collection:
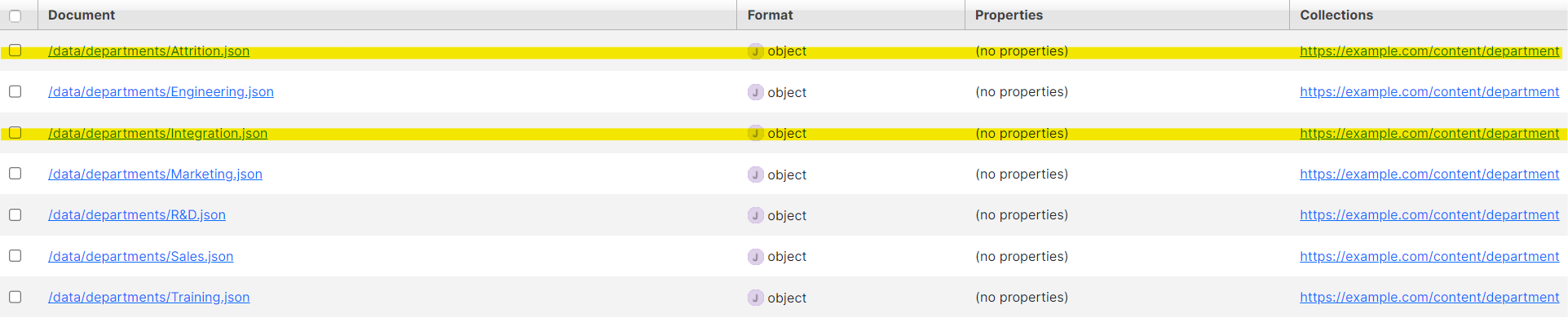
To return rows, use
result()
instead ofexecute()
.You can insert XML documents equivalent to the JSON ones that we inserted above. This example shows the Integration Department document being inserted using
xdmp.unquote()
and the Attrition Department document being inserted usingNodeBuilder
functions:declareUpdate(); const op = require("/MarkLogic/optic"); const collections = ["https://example.com/content/department"]; const permissions = xdmp.defaultPermissions(); const ns = "http://example.com/example" const integrationDoc = xdmp.unquote(` <Department xmlns="http://example.com/example"> <Name>Integration</Name> <LineOfBusiness>Cross-Department Collaboration</LineOfBusiness> <Description>The Integration Department helps the new company determine optimal allocation of resources and employees moving forward.</Description> </Department> `) const attritionDoc = new NodeBuilder() .startElement("Department", ns) .addElement("Name", "Attrition") .addElement("LineOfBusiness", "Cross-Department Collaboration") .addElement("Description", "The Attrition Department contains potentially redundant employees identified as the integration process progresses.") .endElement() .toNode() op.fromDocDescriptors([ { uri: "/data/departments/Integration.xml", doc: integrationDoc, collections, permissions }, { uri: "/data/departments/Attrition.xml", doc: attritionDoc, collections, permissions }]) .write() .execute();